Graphics.h File For C
Creating 2D graphics programs under DOS is easy if you’re using [turbo c]. There is library file called graphics.h that does the tiresome work for you. But unfortunately this library is borland specific you can’t use it on other compilers.
The C graphics.h is the header file which should be included to initalize your computer to start using grahipcs methods and also to initialize the monitor.These kinds of statements before the main program are called preprocessor dierctives.Its very simple as coded below. Initializing Graphics Form Borland C graphics library. Install Dev-C. I installed from the Version 4.9.9.2 Setup File. Download graphics.h to the include/ subdirectory of the Dev-C directories. Download libbgi.a to the lib/ In order to use the WinBGIm subdirectory of the Dev-C directories. Whenever you #include graphics.h in a program, you must instruct the linker to link in certain libraries. I tried ur prg in VC 2008 Express, but it looks like it doesn't support BGI graphics like TC( no 'graphics.h' header file in the 'C: Program Files Microsoft Visual Studio 9.0 VC include' dir ). Currently i don have any other compiler installed on my PC, tommorow i'll try it on my friend's PC and post my solution. About: TurboC is a linkable library and a set of C header files that make it easier to port C code originally written for Borland’s MS-DOS based Turbo C compiler to GNU gcc. Development code. Development code. Download the RAR file from here. Exact all files from the RAR file. Open 'Extra' Folder. Copy the two files Graphic.h and Winbgim.h 4. After successful install, Open Installed file location of CodeBlocks. Make a sure CodeBlocks is closed totally. Default location: for 64bit PC: C: Program Files (x86) CodeBlocks. In order to run graphics programs under Dev-C you have to download WinBGIm files. Download the files listed below. Graphics.h (download to C: Dev-Cpp include) libbgi.a(download to C: Dev-Cpp lib) Once you download the files. Now you have to place into the correct location in Dev-C installation folder. In this article, we will learn the use of ‘graphics.h’ in language C and will also make some programs based on our learning. Submitted by Sneha Dujaniya, on June 28, 2018 Color Description in C. Setbkcolor sets the background to the color specified by the color or the number. The argument color may be a name or a number as given in the.
Even though some peoples somehow managed to port it outside the turbo. Some people hacked their own version of graphics.h. One such person is Micheal main, he ported some of borland graphics functions and library.
Micheal main modified BGI library for windows application to be used under MinGW. This BGI library is renamed as WinBGIm. Now you can use all the borland specific functions under Dev-C++.
Installation
In order to run graphics programs under Dev-C++ you have to download WinBGIm files. Download the files listed below.
- Graphics.h (download to C:Dev-Cppinclude)
- libbgi.a(download to C:Dev-Cpplib)
Once you download the files. Now you have to place into the correct location in Dev-C++ installation folder. Try to locate include and lib folder under your dev-cpp installation. Move these files under the respective folder of include and lib. like e.g. D:Dev-cpp include & D:Dev-cpplib .
Configuration
At last step you’ve downloaded & installed the WinBGIm, now you have to configure it to use under Dev-C++. You’ve to set some project options in Dev-C++ in order to run WinBGIm references properly.
Follow the steps below to set proper project options for WinBGIm.
1. Go to the “File” menu and select “New”, “Project”,Choose “Empty Project” and make sure “C++ project” is selected. Give your project suitable name and click on “Ok”.
OR
1. You can create individual C++” source file” instead of “project”. Go to the “File” menu and select “New Source File” OR Go to the “Project” menu and select “New File”.
2. Go to “Project” menu and choose “Project Options”.
3. Go to the “Parameters” tab.
4. In the “Linker” field, enter the following text: Roland tr 808 vst crack.
- -lbgi
- -lgdi32
- -lcomdlg32
- -luuid
- -loleaut32
- -lole32
5.Click “Ok” to save settings.
Now you’ve done with the configuration for WinBGIm. Please make sure you’ve done this step properly otherwise compiler will flag error.
Testing & Debugging
Now let’s write a small program to test how WinBGIm works. Here is the source code for the program. Type it down,save it with .cpp extension and compile and run to see the results.
#include <graphics.h>
#include <iostream>
using namespace std;
int main()
{
initwindow(800,600);
circle(200,300,600);
while(!kbhit());
closegraph();
return 0;
}
This is the program for displaying circle with respective parameters on window of size 800×600.This window will close when you press any key.If you’ve made settings correctly then you can view the graphics,without any problem.
What’s included ?
All the borland graphics batteries included, plus some additional written by other contributors of WinBGIm. With WinBGIm you can use most of the borlands graphics function & RGB colors. You can also use detectgraph() and initgraph() or you can use new function called initwindow(). You can even use some of the old mouse function such as int mousex() & int mousey() along with getmouseclick() & clearmouseclick(). For keyboard functions,you don’t have to include conio.h some of the functions are supported without it like void delay(int millisec),int getch( ),int kbhit( ).
If you want to capture the screen where you’ve created your graphics. You can do it with help of these functions getimage(),imagesize(), printimage(), putimage(), readimagefile() ,writeimagefile().
Help & Support
If you’re into some trouble with installation & configuration,then please post your questions here. But please don’t post homework problems or your custom projects.Google groups is the right place to get answers in such cases. You can even get lot of support with WinBGIm and Dev-C++ at Google groups. If you want to read about the WinBGIm documentation & FAQ.
If you’ve any question or suggestion then don’t hesitate to post it here.If you know any alternative than WinBGIm,please post about it here.
This page describes gfx, a simple graphics library for CSE 20211.This library is meant to be simple and easy to learn, so that beginningCSE students can get right into the interesting parts of programming.The gfx library only requires that the programmer understandhow to invoke basic C functions with scalar arguments.It is more than enough to explore raster and vector graphics,create animations, draw fractal shapes, and write simple video games. It doesn't do everything that you might want a graphicslibrary to do. As you learn more about programming, more advancedlibraries that you might consider using are OpenGL for precise 3-D graphics, Qt for windowed applications, and SDL for video games.
This library runs on Linux machines using the X11 Window System. (You may be able to run it on a Mac, but it won't work on Windows.) So, you will have to go to the computer lab to work on this assignment.
Getting Started
Download the following files and put them in your lab directory:To compile a program that uses the gfx library, you need touse the following command, which compiles your program with gfx.cthen adds the X11 and math libraries. (X11 is short for the X Window System used on Unix based machines.)
On a Mac with XCode and the X Windows option installed, the following might work:Line by Line Example
To give you the idea of how to use the library, we will point outthe important parts of example.c in detail. Then, belowyou can read about how the individual functions work.Begin by including gfx.h, to make the library available to the program. Note that quotes rather than angle brackets are used in this statement. Quotes indicate the header file is in the current directory, rather than in some system-defined place.
Graphics.h File For Dev C++ Download
gfx_color sets the current drawing color to a pale green:Function Reference
Red | Green | Blue | Color Name |
---|---|---|---|
255 | 0 | 0 | Red |
0 | 255 | 0 | Green |
0 | 0 | 255 | Blue |
255 | 255 | 0 | Yellow |
255 | 255 | 255 | White |
100 | 100 | 100 | Gray |
0 | 0 | 0 | Black |
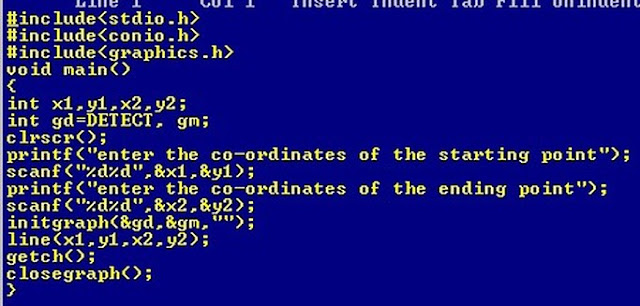